Web scraping is an invaluable technique in today’s data-driven landscape, supporting the collection of structured information from the web. Utilized across various sectors, from market research to academic studies, web scraping serves as a cornerstone in the acquisition of up-to-date and comprehensive data.
Known for its readability and robust library ecosystem, Python is a preferred language for web scraping tasks. Libraries like BeautifulSoup and Selenium simplify the extraction process and provide a range of functionalities tailored to handle diverse website structures. This blog post is an in-depth guide on the usage of Python for web scraping, covering essential libraries, methodologies, and best practices.
What is Web Scraping?
Before getting into the technical know-how, let’s begin with defining web scraping. Web scraping is the process of extracting data from websites, often by making HTTP requests to a specified URL and then parsing the HTML content to retrieve specific data. This data is then typically saved to a database or spreadsheet for further application, from data analysis to competitive intelligence.
Prerequisites for Web Scraping Using Python
To get started with your scraping quest, you'll first need to set up your development environment. The essential tools include Python, a code editor, and relevant Python libraries.
Python: Python's versatility and extensive libraries make it an ideal choice as a programming language. Download Python from its official website.
Code Editor: A reliable code editor is pivotal for writing and debugging your Python scripts. Visual Studio Code is a widely-used editor that offers excellent Python support, but feel free to explore other alternatives like PyCharm and Jupyter Notebook.
Python Libraries: You'll need to install specific libraries that facilitate web scraping, the most popular of which include Beautiful Soup and Selenium. These libraries can be installed using pip, Python's package manager.
Choosing a Web Scraping Library
Selecting the right library is a crucial step in your web scraping project. Below are some of the most popular Python libraries used in the field, each with its own set of advantages and limitations:
Requests
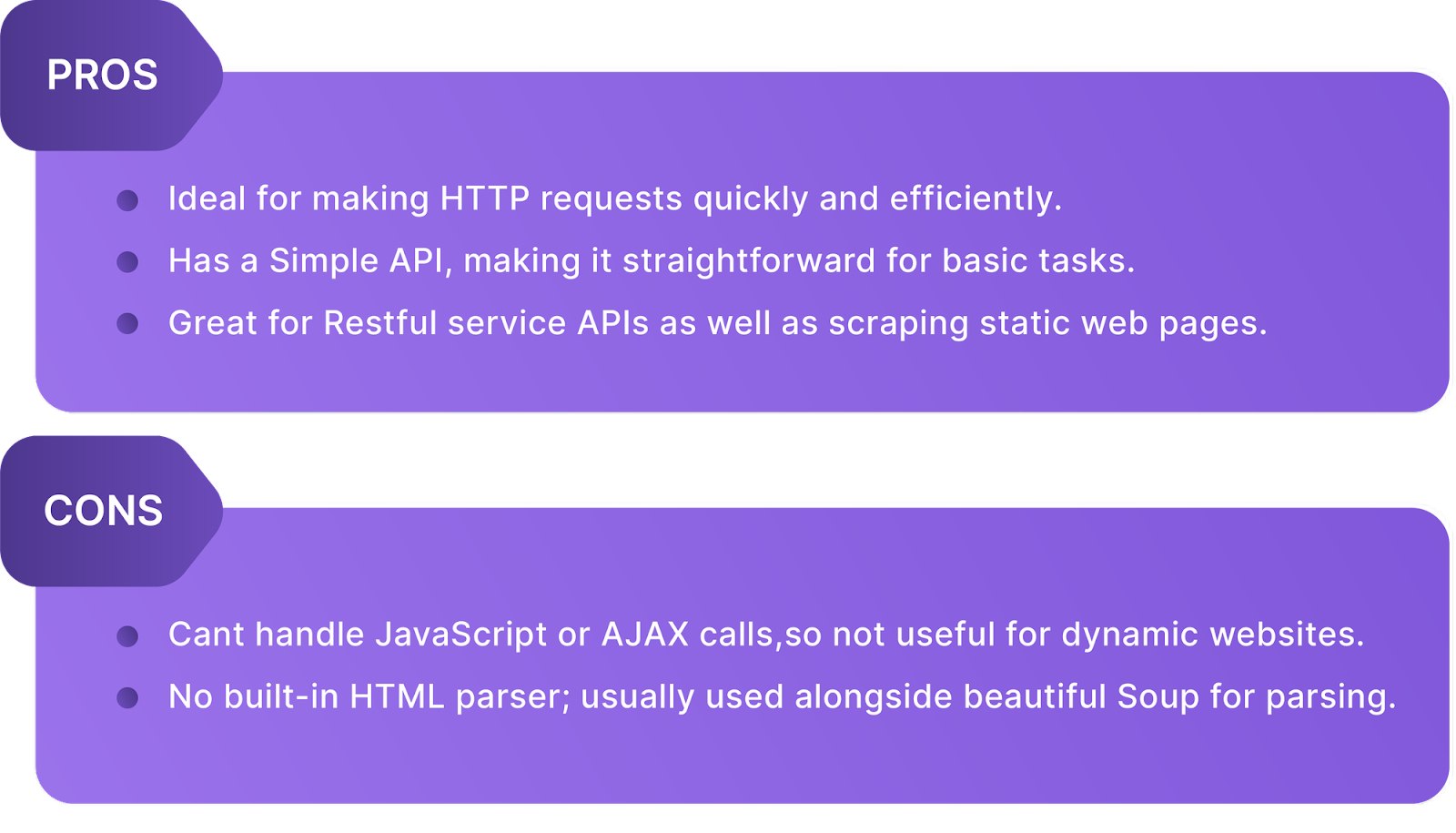
Beautiful Soup
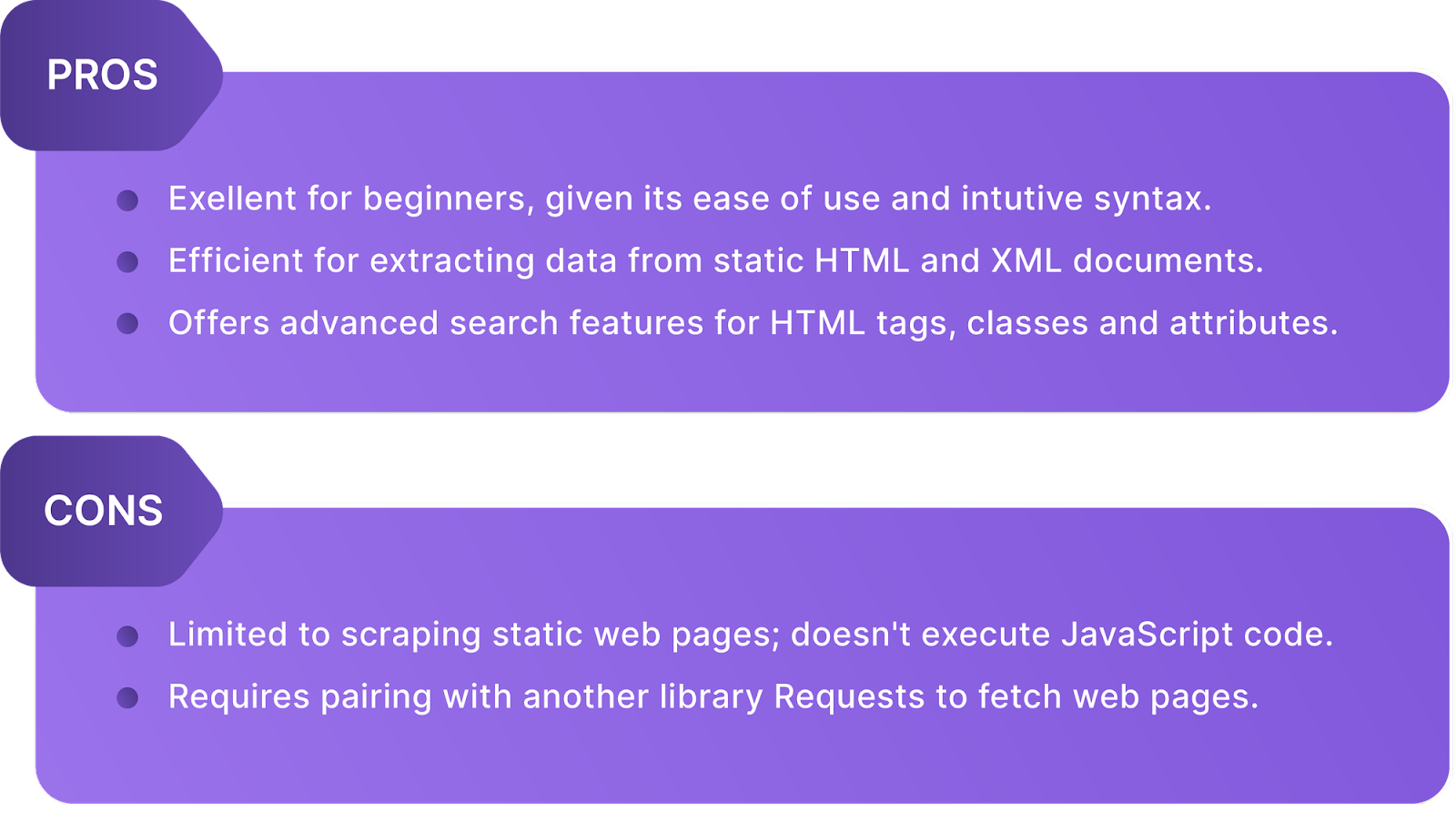
Selenium
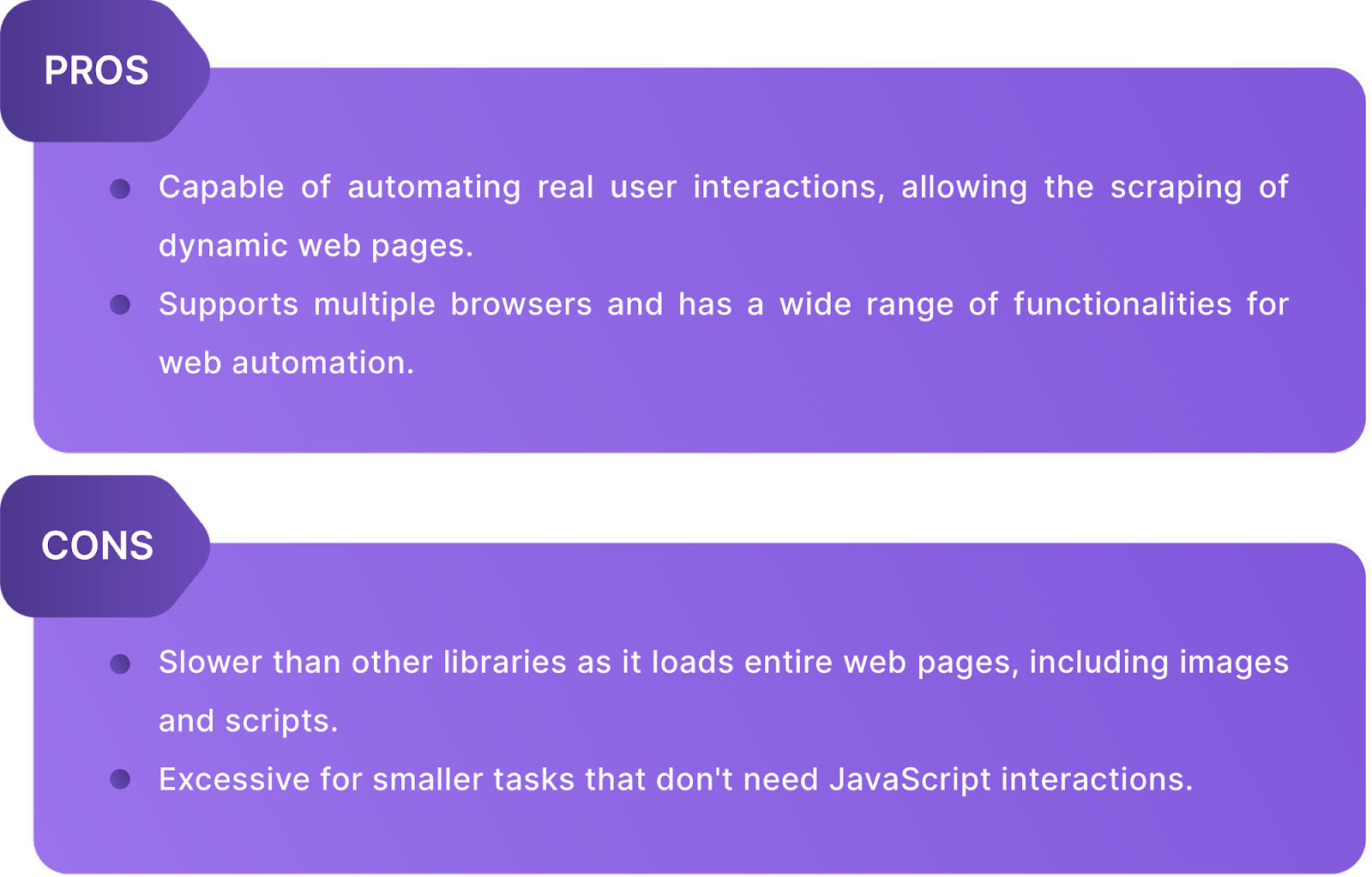
Scrapy
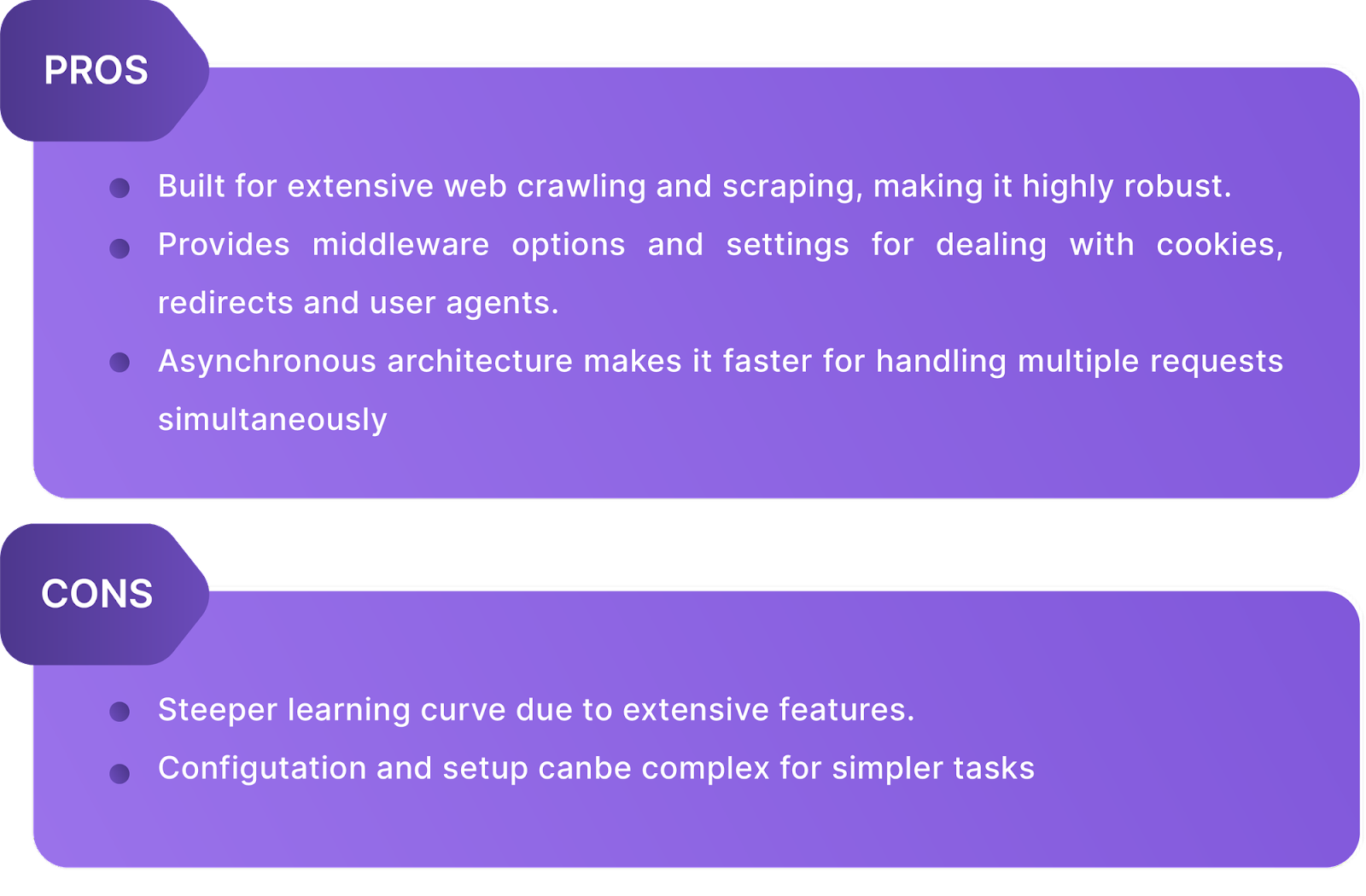
Each library has its own niche, so narrow down your choice based on the specific requirements of your project. Whether you need to scrape static pages, interact with dynamic websites, or even build a full-fledged web crawler, there's a library for you.
Setting Up Your Python Environment
To set up a conducive Python environment, it's crucial to establish a virtual environment and have the necessary libraries in place. You can do it by:
1. Creating a Virtual Environment
Open your terminal and navigate to your project directory. Run the following command to create a virtual environment:
To activate it, use:
2. Installing Libraries
With the virtual environment active, you can then install the libraries. Run:
Importance of Virtual Environments
- They isolate your project dependencies, safeguarding against conflicts with system-wide packages.
- They offer a clean slate, allowing you to install, update, or delete packages without affecting other projects or system settings.
- They help in replicating your setup, making it easier to share your project with others or deploy it to a server.
In summary, virtual environments play a significant role in managing dependencies and ensuring that your project runs smoothly across different setups.
Making HTTP Requests
Fetching web pages is the starting point of any web scraping task, and the Requests library makes this process straightforward. Here's how to use it:
1. Fetching a Web Page with GET Request
2. Handling HTTP Status Codes
Always check the status code before proceeding.
3. Working with Headers
Headers could be important for scraping as they can help bypass certain restrictions.
4. Making a POST Request
POST requests are often used for submitting form data or uploading files.
Understanding how to properly make HTTP requests and handle responses is key to successful web scraping. The Requests library makes it relatively easy to achieve these tasks with its extensive range of functionalities for managing HTTP transactions. Check out the official Requests documentation to learn more about the scope of the library.
Parsing HTML with Beautiful Soup
Beautiful Soup is a Python library designed for web scraping tasks that involve HTML and XML parsing. Its main features include searching, navigating, and modifying the parse tree.
1. Getting Started with Beautiful Soup
To begin, you'll need to install it:
Import the library and load a webpage content into a Beautiful Soup object:
2. Extracting Data
To extract specific data points, you can search for HTML tags, classes, or IDs:
3. Navigating HTML Elements
You can navigate the HTML tree using relationships like .parent, .contents, or .next_sibling:
4. Searching Multiple Elements
Beautiful Soup also allows you to search for multiple elements at once:
Beautiful Soup offers a wide array of functionalities to navigate through an HTML document efficiently. With just a few lines of code, you can extract valuable data from web pages and use it for your projects. Take a look at the official BeautifulSoup documentation to get a better understanding of the code.
Advanced Scraping with Selenium
While Beautiful Soup and Requests are great for static pages, Selenium can step in when you need to scrape dynamic websites that rely heavily on JavaScript. Selenium can automate browser tasks, enabling you to interact with web elements and handle AJAX calls. It's the go-to solution when dealing with complex, interactive web pages.
1. Setting Up Selenium
First, you'll need to install the Selenium package:
Then, download the appropriate WebDriver, like ChromeDriver, from the official site.
2. Basic Interactions
3. Filling Forms
To automate form submissions, you can locate input fields and buttons and interact with them:
4. Handling Dynamic Content
Selenium can wait for elements to load, so you can scrape the data you need:
With Selenium, you can perform advanced web scraping tasks that require form submissions, infinite scrolling, or any other interactive features present in dynamic web pages.
Common Web Scraping Challenges
Web scraping is not always a walk in the park. You might encounter hurdles like CAPTCHAs, AJAX-based loading, and IP blocking. Here's how to tackle them:
- CAPTCHA Handling: Consider using OCR (Optical Character Recognition) tools or third-party services that solve CAPTCHAs. However, automated CAPTCHA solving may violate some websites' terms of service, so use them responsibly and appropriately.
- Managing AJAX Calls: Opt for Selenium when you need to wait for AJAX elements to load. Another method could be sending XHR requests directly, mimicking how the browser retrieves data via AJAX.
- IP Blocking: If you find your IP getting blocked, you could use proxy servers to rotate your IP address. Some libraries can be integrated directly with Python for this purpose.
Strategies for Complex Websites
- Rate Limiting: Throttle your request speed to avoid getting banned.
- User-Agent Rotation: Rotate user agents to make your requests appear more natural.
- Data Storage: For large-scale scraping, consider using databases to store your scraped data.
These strategies and tools can help you significantly improve the efficiency of your web scraping activities while reducing the chances of getting blocked or running into other issues.
Storing Data
Once you’ve scraped the data, the next logical step is to store it in a usable format. Let's explore some commonly used storage options and how you can implement them:
1. CSV (Comma-Separated Values)
Great for tabular data.
Code example:
2. JSON (JavaScript Object Notation)
Ideal for hierarchical or nested data.
Code example:
3. Databases (SQL, NoSQL)
Best for complex or large-scale data that requires relational or distributed storage.
SQL example using SQLite:
Picking the right storage option depends on the scale of your project and the kind of data you're dealing with. Whether it's simple CSV files, hierarchical JSON files, or robust databases, each has its own set of advantages and use-cases.
Troubleshooting and Debugging
Even the best-laid plans can run into snags, and web scraping is no exception. Knowing how to debug effectively can save you a ton of time.
You may come across common issues such as:
- 404 Not Found: When the resource you’re looking for isn't available. Double-check your URLs.
- 403 Forbidden: You might be scraping too fast or need to use headers. Consider rate-limiting or rotating IPs.
- Timeout Errors: These occur when a request takes too long. Adjust the timeout settings in your HTTP requests.
When web scraping using Python, these are some measures readily available within the language for resolving problems:
- Reading Error Messages: Python's error messages often point you right to the issue. Don't ignore them; read carefully to understand what went wrong.
- Handling Exceptions: Use Python’s try-except blocks to tactfully handle errors without crashing your entire script.
- Debugging Tools: Use Python's built-in pdb for debugging or consider using IDE-specific debugging tools.
- Logs and Monitoring: Maintain logs of your scraping activities. They can be invaluable for tracing back what went wrong and when.
Debugging might not be glamorous, but it's essential. The key is to preempt common errors, handle exceptions with presence of mind, and arm yourself with reliable debugging tools.
Best Practices and Ethics in Web Scraping
As powerful as web scraping is, it comes with responsibilities. Ethical considerations should never be an afterthought in any such endeavor.
- Respectful Scraping: Always abide by the website's terms of service. Some websites explicitly forbid scraping, and disregarding this can lead to legal consequences.
- Don't Overload Servers: Sending too many requests in a short period can overload a website's server, affecting its performance. Use rate-limiting to moderate your request speed.
- Robots.txt: This file, usually located at website.com/robots.txt, provides guidelines on what you're allowed to scrape. Ensure you adhere to its directives.
Strategies for Responsible Scraping
- Use a crawl-delay to space out your requests.
- Scrape during off-peak hours to minimize impact on server load.
- Cache pages locally to avoid repeated requests to the same page.
Remember, just because you can scrape a website doesn't mean you should, especially if it could negatively impact the website or violate privacy norms. Practicing ethical scraping is not only the right thing to do, but also crucial for the long-term sustainability of web scraping.
Automating Web Scraping
Once your scraping code is solid, the next step is automating the process. Periodically or individually running code is tedious, and frankly unwarranted. Plus, manual runs won’t cut it if you need up-to-date data. Let’s look at some automation techniques:
1. Cron Jobs: On Linux and macOS systems, you can use the Cron utility to run scripts at scheduled intervals.
2. Windows Task Scheduler: For Windows users, the Task Scheduler can accomplish similar tasks. Just point it to your Python script and set your desired frequency.
3. Cloud-Based Solutions: Services like AWS Lambda can automate your Python scripts in the cloud, making them OS-independent and more resilient.
4. Error Handling and Notifications: Integrate your automation solution with some sort of alerting system. If something fails, you should know about it immediately.
Automating your web scraping tasks frees you from the need to manually trigger your scripts, ensuring you have the latest data when you need it. Pair this with good error handling, and you've got yourself a robust, self-sufficient data gathering mechanism.
An Easier Alternative to Web Scraping With Python
Since we just went over web scraping automation, there’s a simpler and quicker way to extract online data. Especially if coding isn't your forte, Browse AI offers a seamless, no-code solution to web scraping. With its user-friendly point-and-click interface, you can train a custom robot or use a pre-built robot to automate data extraction from websites—in just 2 minutes. Plus, the platform is versatile enough to turn websites into spreadsheets or APIs, or even create streamlined workflows by integrating with Airtable, Zapier, Make.com, and many other popular applications.
Final Words
Web scraping is a powerful technique for extracting valuable data from the web, and Python offers a plethora of tools to make it accessible and efficient. From choosing the right libraries like Beautiful Soup and Selenium to setting up your environment and handling common challenges, we hope this guide covered all bases. And for those who prefer a no-code solution, Browse AI is a highly efficient alternative worth exploring. The key to mastering web scraping lies in practice and continuous learning. So, what are you waiting for? Dive in, start scraping, and unlock a world of data waiting for you.
Additional Resources
Here are some additional resources to help you on your web scraping journey:
- Official Python website
- Beautiful Soup Documentation
- Requests Documentation
- Scrapy Documentation
- Selenium Documentation
Feel free to explore these resources to expand your knowledge and tackle more complex projects.